TensorFlow Ops
一般流程:
TFboy基操:
- 定义图
- 创建writer,两种方式
- tf.get_default_graph()
- sess.graph
- 创建session执行图
- 关闭writer
- TensorBoard可视化
1 | import tensorflow as tf |
writer会将图(包含的各种ops)以日志文件的形式写入指定目录,tensorboard可以将这些文件可视化出来
上面的程序每跑一次就会产生一个日志文件,不用的日志及时删除
1 | tensorboard --logdir='./graphs/low' --port=6006 |
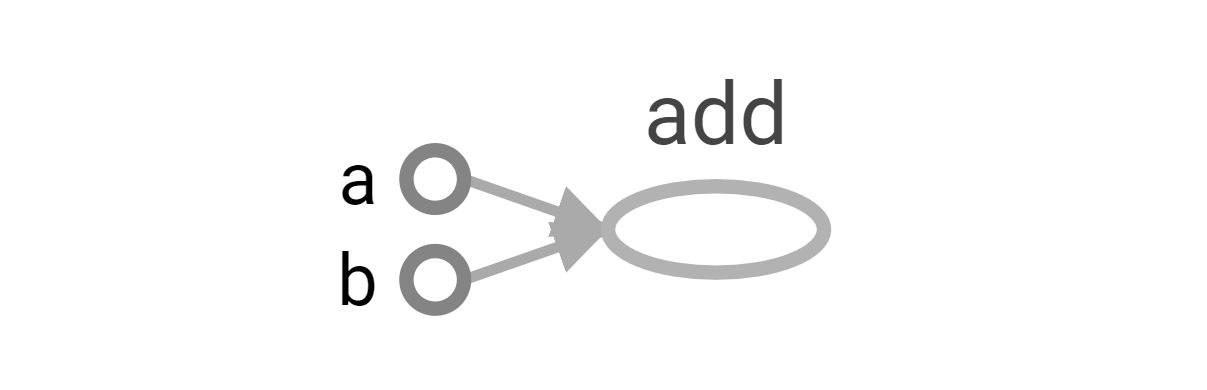
没记住的
1 | tf.fill([2,3],8) ===> [[8,8,8],[8,8,8]] |
变量
变量定义
训练中需要更新的参数定义为变量
常量存储在图中,变量则可能在parameter server上
常量占了很多存储时,加载图会很慢
1 | # old way |
变量初始化
1 | #一次性初始化所有变量 |
变量的值
1 | # 两种方式 |
变量赋值
1 | # 赋值会完成初始化的工作 |
控制依赖
1 | # g has 5 ops: a,b,c,d,e |
数据导入
old way:placeholders and feed_dict
例如,对于f(x,y) = 2x + y,x y 就是真实值的占位符
1
2
3
4
5
6
7
8
9
10
11
12#shape=None 意味着接收任意shape的张量
tf.placeholder(dtype,shape=None,name=None)
#any tensors that are feedable can be fed
tf.Graph.is_feedable(tensor)
#feed_dict可以用来测试模型,
#直接传入某些值免去了大量的计算
a = tf.add(2, 5)
b = tf.multiply(a, 3)
with tf.Session() as sess:
print(sess.run(b)) # >> 21
# compute the value of b given the value of a is 15
print(sess.run(b, feed_dict={a: 15})) # >> 45new way: tf.data
[ ] todo…
lazy loading
需要计算op的时候才创建
因为训练绝大数情况都要计算多次,所以lazy loading会产生大量的冗余节点
解决办法:
- 尽可能的将ops的定义和计算分开(不要在计算的时候创建op
- 当你将相关ops组合在一起(比如,1中的步骤在一个类里面)可以使用python的@property机制确保某些功能只执行一次(!!!有待研究)